Lab 24
Text File Input/Output
CS211 Lab Policy:
- This lab exercise will not be graded.
- Submit as much as you have completed before the end of the lab period in
which it is assigned.
- If you do not finish this lab work, it is to your advantage to finish it
outside of class. Please re-submit your finished work to the course web
site.
- You may receive help from anyone in completing this lab.
- You may not submit another student's code as part of your
lab.
Instructions:
For this lab you will create one MATLAB program named Lab24()
in file Lab24.m. In this assignment you will read statistics about the
2007 NCAA Basketball season (source:
http://web1.ncaa.org/stats/StatsSrv/rankings?sportCode=MBB&rpt=wkly
). Download the file NCAA Basketball
Stats.zip and save it in a new empty folder in your MATLAB working directory. After you have downloaded the file, use a "My Documents" window to navigate to
the folder that you saved the file in. Then right-click the
"NCAA Basketball Stats.zip" file and select
"Extract Here". You should see 15 data files appear in this folder. You will
read data from these files to accomplish this lab assignment.
Implement MATLAB code that does the following. Save your
Lab24.m file in the same directory as
your data files.
Task 1: Determine the statistics for which Air Force is nationally
ranked.
- Clear the command window.
- Use the MATLAB dir('*.txt')
command to get a list of all the text files in the current folder. If you
followed the instructions above and saved the downloaded zip file in an
empty folder, this command will return you a list of all the statistics file
names in a structure array. Experiment with this command in the command
window before you use it in your program.
- For each of the statistic files, do the following:
Action |
MATLAB hints |
Open the file for reading. |
File_id = fopen() |
While the file has not been totally read |
while ~ feof(File_id) |
Read a single line from the file |
Use fgetl() |
Search the line for the string 'Air Force' |
Use strfind() |
if 'Air Force' is found |
|
Extract the first
number from the line of text |
Use sscanf() |
Display a message
that contains the rank and the file name |
|
Close the file |
Use fclose() |
This section of your code should produce output similar to the text shown
below (2006 data shown below):
Air Force is Nationally ranked in Basketball in the following categories:
Rank Category
11 Field-Goal Percentage.txt
22 Free-Throw Percentage.txt
10 Personal Fouls Per Game.txt
1 Scoring Defense.txt
19 Scoring Margin.txt
14 Three-Point Field Goals Per Game.txt
6 Three-Point Field-Goal Percentage.txt
5 Turnovers Per Game.txt
11 Won-Lost Percentage.txt
Task 2: Manipulate the 'Scoring Defense' statistics.
- Create a copy of the file 'Scoring Defense.txt'
and call the new file 'Scoring Defense without
headers.txt'. Then open this new file, delete the first 5 lines of
the file, and save the file. This creates a file that is completely in table
format.
- Use the MATLAB textread() command to read the
'Scoring Defense without headers.txt' statistics
file into
a set of parallel arrays. Use the following format specifiers
in your textread() command.
- Use %d to read the team rank
(1st column)
- Use %20c to read the team
name (2nd column). This will create a 2D array of characters. (You
cannot use %s because some of the
team names include spaces, and %s
reads characters up to the first space.)
- Use %d to read the number of
games played (3rd column).
- Use %d to read the number of
games won (4rd column).
- Use %*c to read the single
slash character between the number of games won and lost (5th column).
(But ignore the slash!)
- Use %d to read the number of
games lost (6th column).
- Use %d to read the total
number of points scored by their opponents (7th column).
- Use %f to read the percentage
total number of points scored by their opponents (8th column).
- Produce a line graph of the total number of points scored by each team's
opponents. The graph should look like (2006 data shown below):
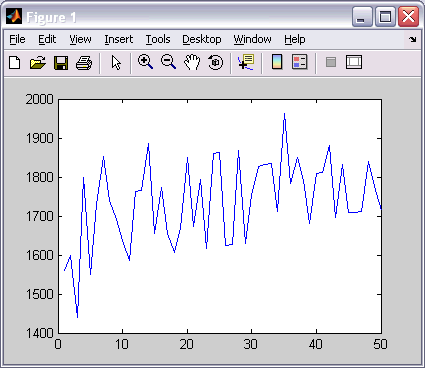
Task 3: Manipulate the original 'Scoring Defense' statistics.
- Now use textscan() to
read the original 'Scoring Defense.txt' data
file. To do this, you must read the first 5 lines of the file before using
textscan(). Perform the following
steps:
- Open the file using fopen().
- Read the first 5 lines using fgetl().
- Read the entire table of data into a single cell array using
textscan().
- Close the file using fclose().
- Produce a scatter plot that compares the number of games won verses the
number of games lost. The graph should look like (2006 data shown below):
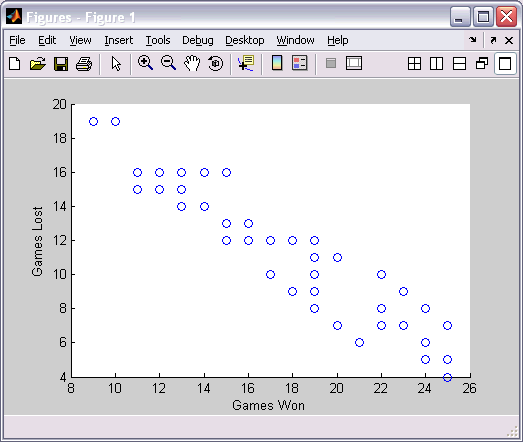
Please make sure you understand the differences and similarities between the
textscan() and the
textread() commands.
Turn-in:
Submit your
Lab24.m file.